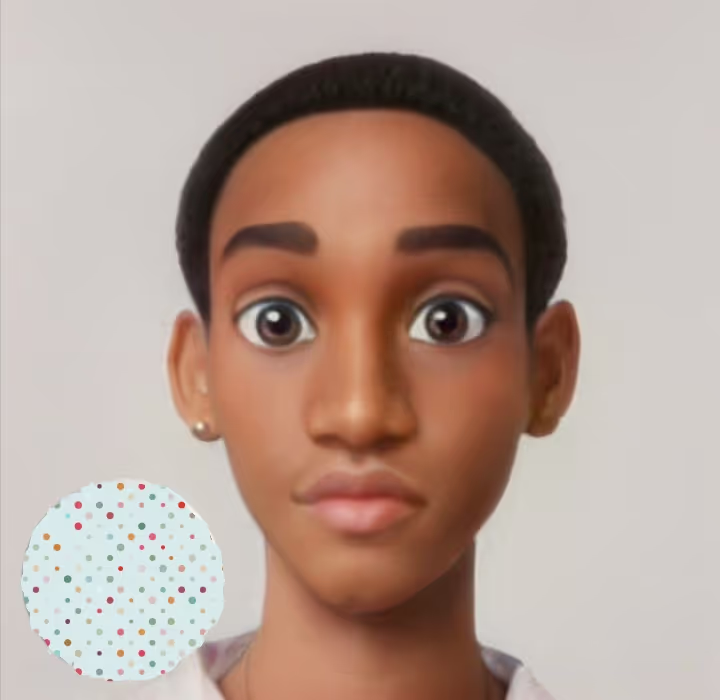
How to Group Routes in Golang Echo
Grouping routes is one of the most important features of a web framework as it helps to organize the routes in a better way. It also allows you to apply specific middleware to a specific group of routes. For instance in a eccomerce website, you might want to apply an authentication middleware to all the routes related to the cart checkout but none to products. In this article, we will learn how to group routes in Golang Echo.
Prerequisites
I expect that you already have a basic understanding of Golang and Echo. If not, you can check out my previous articles on Golang Echo Tutorial.
Grouping Routes
To group routes, we use the Group()
method of the Echo
instance.
package main
import (
"log"
"github.com/labstack/echo/v4"
)
func main() {
e := echo.New()
// general routes
e.GET("/", func(c echo.Context) error {
return c.String(200, "Something is happening")
})
// grouped routes
group := e.Group("/api")
group.GET("/products", func(c echo.Context) error {
return c.String(200, "Products")
})
group.GET("/cart", func(c echo.Context) error {
return c.String(200, "Cart")
})
group.GET("/checkout", func(c echo.Context) error {
return c.String(200, "Checkout")
})
e.Logger.Fatal(e.Start(":1323"))
}
In the above program we have grouped the routes under the /api
path. So, the routes will be accessible at /api/products
, /api/cart
and /api/checkout
.
To add a middleware to a group of routes, we can use the Use()
method of the Group
instance.
...
// grouped routes
group := e.Group("/api")
group.Use(func(next echo.HandlerFunc) echo.HandlerFunc {
return func(c echo.Context) error {
fmt.Println("Middleware for /api")
return next(c)
}
})
...
We can also have nested routes by calling the Group()
method on the Group
instance.
...
// grouped routes
group := e.Group("/api")
group2 := group.Group("/v1")
group2.GET("/products", func(c echo.Context) error {
return c.String(200, "Products")
})
This will create a nested route at /api/v1/products
.
Conclusion
Thats it for this article. In this article, we learned how to group routes in Golang Echo.