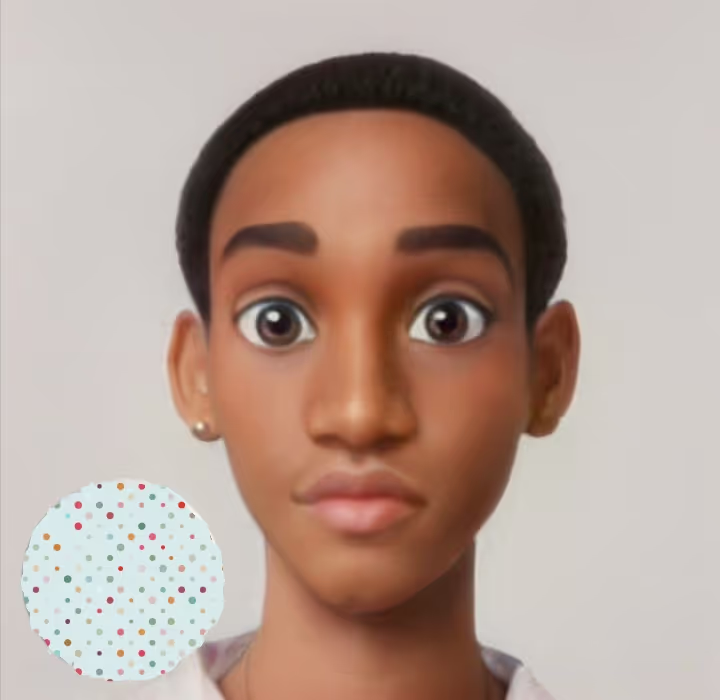
How to convert byte array to string in Golang
In Go, you can convert a byte array to a string using the string()
function. This function takes a byte array as an argument and returns a string. You can also use the fmt.Sprintf()
function to convert a byte array to a string.`
Convert byte array to string using string()
The string()
function takes a byte array as an argument and returns a string. The following example shows how to convert a byte array to a string using the string()
function.
package main
import "fmt"
func main() {
byteArray := []byte{0x68, 0x65, 0x6c, 0x6c, 0x6f}
str := string(byteArray)
fmt.Println(str)
}
The output of the above program is:
hello
package main
import "fmt"
func main() {
byteArray := []byte{'h', 'e', 'l', 'l', 'o'}
str := string(byteArray[:])
fmt.Println(str)
}
The output of the above program is:
hello
Convert byte array to string using fmt.Sprintf()
The fmt.Sprintf()
function takes a format string and a list of arguments as arguments and returns a string. The following example shows how to convert a byte array to a string using the fmt.Sprintf()
function.
package main
import "fmt"
func main() {
byteArray := []byte{0x68, 0x65, 0x6c, 0x6c, 0x6f}
str := fmt.Sprintf("%s", byteArray)
fmt.Println(str)
}
The output of the above program is:
hello
Convert byte array to string using a slice of bytes
You can also convert a byte array to a string by slicing the byte array.
package main
import "fmt"
func main() {
byteArray := []byte{0x68, 0x65, 0x6c, 0x6c, 0x6f, 0x20, 0x77, 0x6f, 0x72, 0x6c, 0x64}
str := string(byteArray[6:])
fmt.Println(str)
}
The output of the above program is:
world
Conclusion
In this article, we learned how to convert a byte array to a string in Go. We learned how to convert a byte array to a string using the string()
function, the fmt.Sprintf()
function, and by slicing the byte array.