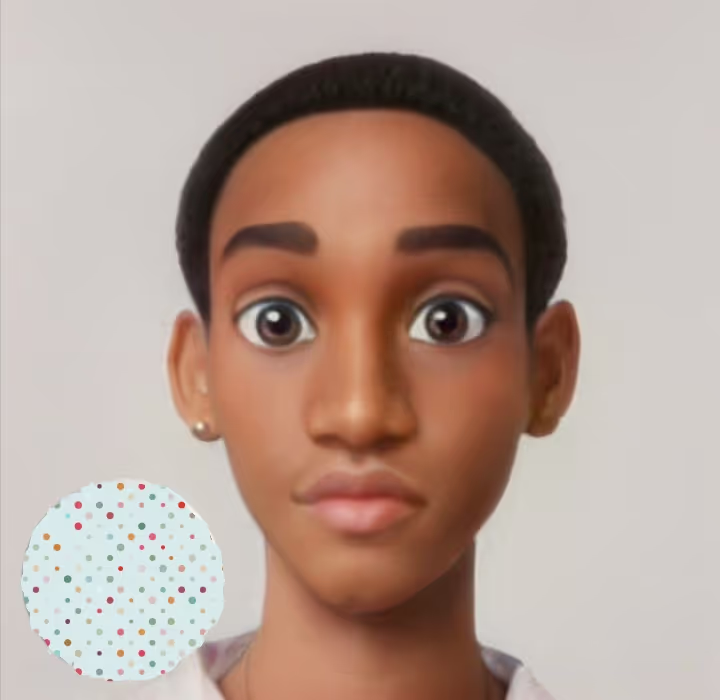
Golang Enum (A Complete Guide)
What is Enum in Golang?
Enum is a type that consists of a set of named constants they can also be referred to as enumarated constants. Constants are used to represent a fixed value that the program may not alter during its execution.
How to Define Enum in Golang
In Go, we can define an enum using the const
keyword. The const
keyword is used to declare a constant value. They are declared outside the function and can be used anywhere in the program.
The following is an example of how to define an enum in Golang.
...
const (
value1 = 1
value2 = 2
value3 = 3
)
...
In the above example, we have defined an enum with three values value1
, value2
, and value3
.
How to Use Enum in Golang
We can use the enum values in the program by using the const
keyword. The following is an example of how to use an enum in Golang.
...
const (
value1 = 1
value2 = 2
value3 = 3
)
func main() {
fmt.Println(value1)
fmt.Println(value2)
fmt.Println(value3)
}
...
In this example, we have used the enum values in the main
function.
Enums and Iota
The iota
keyword is a predeclared identifier in Golang. It is used to represent successive untyped integer constants. It is used to generate a sequence of integer constants. The sequence starts at zero and increments by one.
The following is an example of how to use iota
to define an enum.
...
const (
value1 = iota + 1
value2
value3
)
func main() {
fmt.Println(value1)
fmt.Println(value2)
fmt.Println(value3)
}
output:
1
2
3
In the above instance value1
is assigned the value 1
and the rest automatically increment by one.
How to iterate over an Enum in Golang
To iterate over an enum in Golang, we can use the for
loop. The following is an example of how to iterate over an enum in Golang.
...
const (
value1 = iota + 1
value2
value3
)
func main() {
for i := value1; i <= value3; i++ {
fmt.Println(i)
}
}
By using iota
we generated a sequence of integer constants. We can use the for
loop to iterate over the sequence.
To skip a value in the sequence, we can use the _
operator.
...
const (
value1 = iota + 1
value2
_ // skip value3
value4
)
...
The above example will skip the value 3
in the sequence.
Enum string values
We can assign string values to an enum. The following is an example of how to assign string values to an enum.
...
const (
value1 = "value1"
value2 = "value2"
value3 = "value3"
)
func main() {
fmt.Println(value1)
fmt.Println(value2)
fmt.Println(value3)
}
output:
value1
value2
value3
golang enum type
We can also define an enum type in Golang. The following is an example of how to define an enum type in Golang.
...
type EnumType int
const (
value1 EnumType = iota + 1
value2
value3
)
func main() {
fmt.Println(value1)
fmt.Println(value2)
fmt.Println(value3)
}
output:
1
2
3
In the above example, we have defined an enum type EnumType
and assigned the values value1
, value2
, and value3
to it
Enum Example in Golang
The following is an example of how to use an enum in Golang.
package main
import "fmt"
type Subject int
const (
English Subject = iota + 1
Geography
Mathematics
Physics
Chemistry
Biology
)
// String returns the string representation of the Subject
func (s Subject) String() string {
return [...]string{"English", "Kiswahili", "Mathematics", "Physics", "Chemistry", "Biology"}[s-1]
}
// Index returns the index of the Subject
func (s Subject) Index() int {
return int(s)
}
func main() {
subject := Biology
fmt.Println(subject) // Biology
fmt.Println(subject.Index()) // 6
fmt.Println(subject.String()) // Biology
}
output:
Biology
6
Biology
We have defined an enum type Subject
and assigned the values English
, Geography
, Mathematics
, Physics
, Chemistry
, and Biology
to it. We have also defined two methods String
and Index
to return the string representation and the index of the subject respectively.
Tl;dr
- Enum is a type that consists of a set of named constants that can also be referred to as enumarated constants.
- We can define an enum using the
const
keyword.