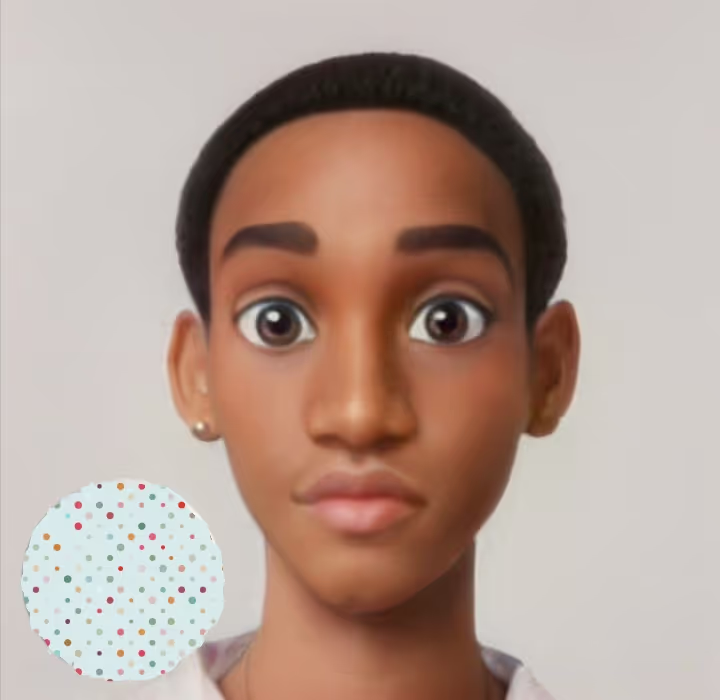
Golang Switch Statements (A Complete Guide)
What is Switch Statement in Go?
The switch statement in Go is a control flow statement that is used to execute a block of code among many alternatives. Switch statements are declared using the switch
keyword followed by the condition to be evaluated. The condition can be a variable, constant, or expression.
The switch
statement is similar to the if-else
statement. The difference is that the switch
statement can have multiple cases. The switch
statement is also known as the switch-case
statement.
Syntax of Switch Statement in Go
The syntax of the switch
statement in Go is as follows:
switch condition {
case value1:
// code block
default:
// code block
}
The switch
statement is followed by the case
keyword. The case
keyword is followed by the value to be compared with the condition. The case
keyword is followed by the code block to be executed if the condition is equal to the value. The default
keyword is used to execute a block of code if none of the cases is true.
Example of Switch Statement in Go
The following example shows the use of the switch
statement in Go:
package main
import "fmt"
func main() {
var num int = 10
switch num {
case 10:
fmt.Println("Number is 10")
default:
fmt.Println("Number is not 10 or 20")
}
}
The output of the above program is:
Number is 10
Switch Statement with Multiple Cases
The switch
statement can have multiple cases, you can have as many cases as you want. The following example shows the use of the switch
statement with multiple cases:
...
switch num {
case 20:
fmt.Println("Number is 10")
case 20:
fmt.Println("Number is 20")
default:
fmt.Println("Number is not 10 or 20")
}
The output of the above program is:
Number is 20
Switch Statement with Fallthrough
Fallthrough is a keyword that is used to execute the next case in a switch
statement. The fallthrough
keyword is used to execute the next case even if the condition is not true. The following example shows the use of the fallthrough
keyword:
...
switch num {
case 10:
fmt.Println("Number is 10")
fallthrough
case 20:
fmt.Println("Number is 20")
default:
fmt.Println("Number is not 10 or 20")
}
The output of the above program is:
Number is 10
Number is 20
In the above example, case 10 is executed and then the fallthrough
keyword is used to execute the next case which is case 20.
golang switch case break
The break
keyword is used to terminate the switch
statement when a given condition is true.
The following example shows the use of the break
keyword:
...
num := 10
switch num {
case 10:
break
fmt.Println("Number is 10")
case 20:
fmt.Println("Number is 20")
default:
fmt.Println("Number is not 10 or 20")
}
The output of the above program is:
Nothing is printed because the switch statement is terminated after the break
keyword.
golang switch channel
A channel is a communication mechanism that allows one goroutine to pass values of a specified type to another goroutine.
The following example shows the use of the switch
statement with a channel:
package main
import (
"fmt"
"time"
)
func main() {
c1 := make(chan string)
c2 := make(chan string)
go func() {
time.Sleep(time.Second * 1)
c1 <- "one"
}()
go func() {
time.Sleep(time.Second * 2)
c2 <- "two"
}()
for i := 0; i < 2; i++ {
select {
case msg1 := <-c1:
fmt.Println("received", msg1)
case msg2 := <-c2:
fmt.Println("received", msg2)
}
}
}
The output of the above program is:
received one
received two
In the above example, the select
statement is used to receive the values from the channel. The select
statement is used to receive the values from the channel. The select
statement is used to receive the values from the channel.
Switch Statement with Multiple Conditions
The switch
statement can have multiple conditions using the ||
, &&
, and !
operators. The following example shows the use of the switch
statement with multiple conditions:
...
num := 10
x := 20
switch {
case num == 10 && x == 20:
fmt.Println("Number is 10 and x is 20")
case num == 20 && x == 20:
fmt.Println("Number is 20 and x is 20")
default:
fmt.Println("Number is not 10 or 20")
}
The output of the above program is:
Number is 10 and x is 20
In the above example, the switch
statement is used to check if the num
variable is equal to 10 and the x
variable is equal to 20.
Switch Statement with Type Switch
The switch
statement can be used to check the type of a variable using the type
keyword. By combining type
and :=
we can create a type switch guard . The following example shows the use of the switch
statement with the type
keyword:
package main
import "fmt"
func main() {
var x interface{}
switch i := x.(type) {
case nil:
fmt.Printf("x's type: %T\n", i)
case int:
fmt.Printf("x is int\n")
case float64:
fmt.Printf("x is float64\n")
case func(int) float64:
fmt.Printf("x is func(int)\n")
case bool, string:
fmt.Printf("x is bool or string\n")
default:
fmt.Printf("don't know the type\n")
}
}
The output of the above program is:
x's type: <nil>
if we change the value of the x
variable to 10
the output will be:
x is int
Tl;dr
- The
switch
statement is used to execute a block of code based on the value of a variable. - The
switch
statement can have multiple cases, you can have as many cases as you want. - The
switch
statement can have multiple conditions using the||
,&&
, and!
operators. - The
switch
statement can be used to check the type of a variable using thetype
keyword.