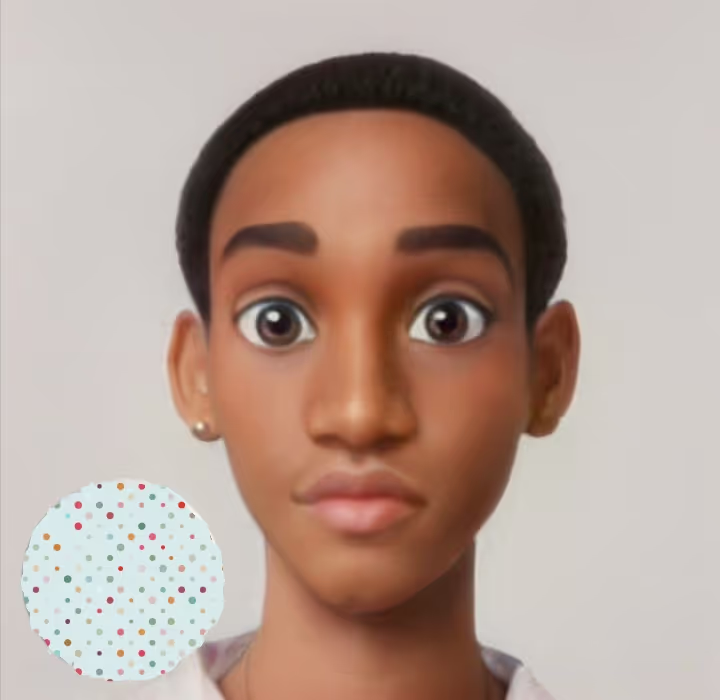
Kevin Kelche
How to measure execution time in Go
How to measure execution time in Go
To measure execution time in Golang we use time.Now()
and time.Since()
functions.
package main
import (
"fmt"
"time"
)
func main() {
start := time.Now()
time.Sleep(2 * time.Second)
elapsed := time.Since(start)
fmt.Println("Time elapsed: ", elapsed)
}
Output:
Time elapsed: 2.0020002s
We can also use time.Now().Sub()
function to measure execution time.
package main
import (
"fmt"
"time"
)
func main() {
start := time.Now()
time.Sleep(2 * time.Second)
elapsed := time.Now().Sub(start)
fmt.Println("Time elapsed: ", elapsed)
}
Output:
Time elapsed: 2.0020002s
We can also use time.Now().UnixNano()
function to measure execution time.
// ....
func main () {
start := time.Now().UnixNano()
time.Sleep(2 * time.Second)
elapsed := time.Now().UnixNano() - start
fmt.Println("Time elapsed: ", elapsed)
}
Output:
"Time elapsed: 2002000000"
A better way to measure execution time of a function is to use the testing package and perfom a benchmark test.
package main
import (
"fmt"
"time"
)
func myFunction() {
time.Sleep(2 * time.Second)
}
func main(){
fmt.Println("Hello World")
}
package main
import (
"testing"
)
func BenchmarkMyFunction(b *testing.B) {
for i := 0; i < b.N; i++ {
myFunction()
}
}
On running the tests we get the execution time of the function after running b.N
times which is the number of iterations.
Learn more about Benchmarking in this article.