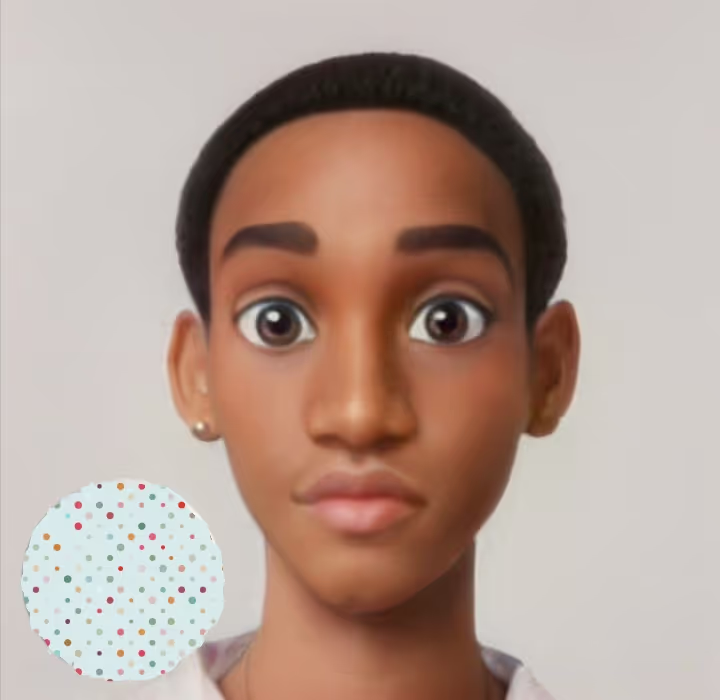
Kevin Kelche
How to print structs in Go
How to print structs in Go
To print structs we use the fmt package i.e fmt.Printf(ā%vā, structName). This will print the struct in the format of a map. To print the struct in a more readable format we use the %#v format specifier. This will print the struct in the format of a struct.
package main
import "fmt"
type Mystruct struct {
Name string
Age int
}
func main() {
myStruct := Mystruct{
Name: "John",
Age: 20,
}
fmt.Printf("%v", myStruct)
fmt.Printf("%#v", myStruct)
}
Output:
{John 20}
main.Mystruct{Name:"John", Age:20}
we can also convert the struct to JSON and then print the elements of the struct
package main
import (
"encoding/json"
"fmt"
)
type Mystruct struct {
Name string
Age int
}
func main() {
myStruct := Mystruct{
Name: "John",
Age: 20,
}
json, err := json.Marshal(myStruct)
if err != nil {
fmt.Println(err)
}
fmt.Println(string(json))
}
Output:
{"Name":"John","Age":20}
How to print a nested struct in Go
To print a nested struct we use the %#v format specifier. This will print the struct in the format of a struct.
package main
import "fmt"
type Mystruct struct {
Name string
Age int
}
type Mystruct2 struct {
Name string
Age int
Mystruct
}
func main() {
myStruct := Mystruct2{
Name: "John",
Age: 20,
Mystruct: Mystruct{
Name: "John",
Age: 20,
},
}
fmt.Printf("%#v", myStruct)
Output:
main.Mystruct2{Name:"John", Age:20, Mystruct:main.Mystruct{Name:"John", Age:20}}
How to print a struct with a slice in Go
To print a struct with a slice we use the %#v format specifier. This will print the struct in the format of a struct.
package main
import "fmt"
type Mystruct struct {
Name string
Age int
}
type Mystruct2 struct {
Name string
Age int
Mystruct
MySlice []string
}
func main() {
myStruct := Mystruct2{
Name: "John",
Age: 20,
Mystruct: Mystruct{
Name: "John",
Age: 20,
},
MySlice: []string{"one", "two"},
}
fmt.Printf("%#v", myStruct)
}
Output:
main.Mystruct2{Name:"John", Age:20, Mystruct:main.Mystruct{Name:"John", Age:20}, MySlice:[]string{"one", "two"}}