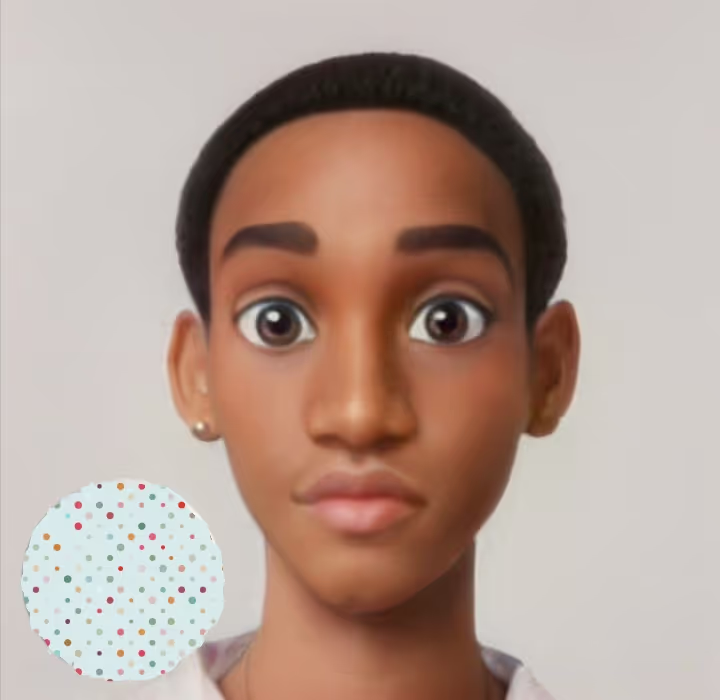
How to accept user input with spaces in Golang
Introduction
When you are building a command line application in Golang, you’ll often need to accept user input. These inputs can sometimes contain spaces. For example, your program is a bookstore search engine. You want to accept a search query from the user. The user might enter something like “The Lord of the Rings”. If you’re using the conventional fmt.Scan...
functions, you’ll only get the first word unless you use a loop to read the rest of the input. In this tutorial, we’ll learn how to accept user input with spaces in Golang.
package main
import (
"fmt"
"log"
)
func main() {
fmt.Println("Enter a title: ")
var title string
_, err := fmt.Scan(&title)
if err != nil {
log.Fatal(err)
}
fmt.Println("You entered: ", title)
}
By running the above program, you’ll see that only the first word is read. The rest of the input is ignored.
❯ go run main.go
Enter a title:
Lord of the rings
You entered: Lord
❯ of the rings
zsh: command not found: of
As you can see in the above example, the program only reads the first word. The rest of the input is ignored and even causes errors in the terminal.
Reading user input with spaces
To read user input with spaces, we’ll use the bufio
package. This package provides a buffered reader that can read a line of input from the command line.
package main
import (
"bufio"
"fmt"
"os"
)
func main() {
reader := bufio.NewReader(os.Stdin)
fmt.Print("Enter a title: ")
title, _ := reader.ReadString('\n')
fmt.Println("You entered: ", title)
}
The above program reads a line of input from the command line and prints it to the terminal. The ReadString
function reads a line of input from the command line and stops when it encounters the specified delimiter. In this case, we’re using the newline character \n
as the delimiter.
$ go run main.go
Enter a Title: The Lord of the Rings
You entered: The Lord of the Rings
$
Unlike the fmt.Scan
function, the bufio
package reads the entire line of input. This means that the user can enter as many words as they want.
This is but one of the powers of the bufio
package. You can learn more from this Golang bufio tutorial.